MERN Stack with Mongo Atlas
*requires signup for Atlas instance*
echo "please give your new MERN app a snake-case name:" && read APP_NAME && mkdir $APP_NAME && cd $APP_NAME && mkdir server && cd server && npm init -y && npm install express cors dotenv && cd .. && echo 'const express = require("express");\n\nconst app = express();\n\nconst cors = require("cors");\n\nrequire("dotenv").config({ path: "./config.env" });\nconst port = process.env.PORT || 5000;\n\napp.use(cors());\n\napp.use(express.json());\n\napp.use(require("./routes/record"));\n\n// Get MongoDB driver connection\nconst dbo = require("./db/conn");\n \napp.listen(port, () => {\n // Perform a database connection when server starts\n dbo.connectToServer(function (err) {\n if (err) console.error(err);\n \n });\n console.log(`Server is running on port: ${port}`);\n});\n' > server/server.js && git add . && git commit -m "basic MERN stack" && cd server && npm install mongoose &&
NextJS Stack
echo "please give your new NextJS app a snake-case name:" && read APP_NAME && npx create-next-app@latest $APP_NAME --use-npm --example "https://github.com/vercel/next-learn/tree/master/basics/learn-starter" && cd $APP_NAME && rm pages/index.js && rm styles/Home.module.css && echo "export default function Home() {\n return (\n <>Hello Next JS</>\n )\n}" > pages/index.js && git add . && git commit -m "new Next JS app"
Start your new app with yarn run dev
Add Cypress to a new NextJS App
yarn add cypress -D && sed -i '' -e 's/"scripts": {/"scripts": {\n "cypress:open": "cypress open",\n "cypress:run": "cypress run",/g' package.json && yarn run cypress open
Go through the initial Cypress steps, choose “E2E Testing” and then “Chrome”
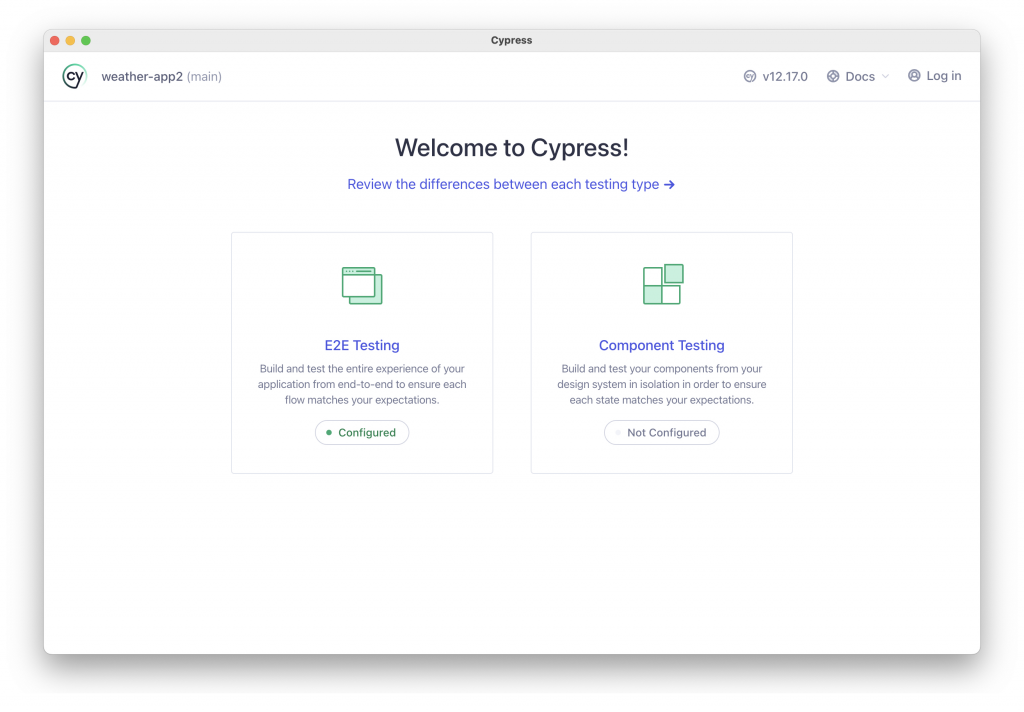
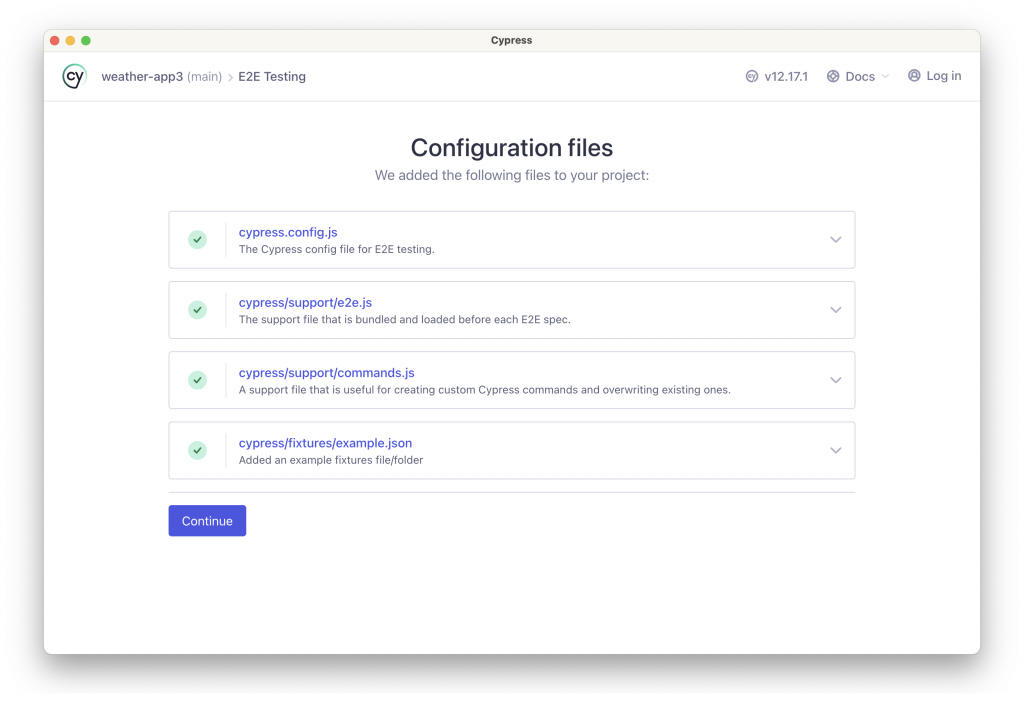
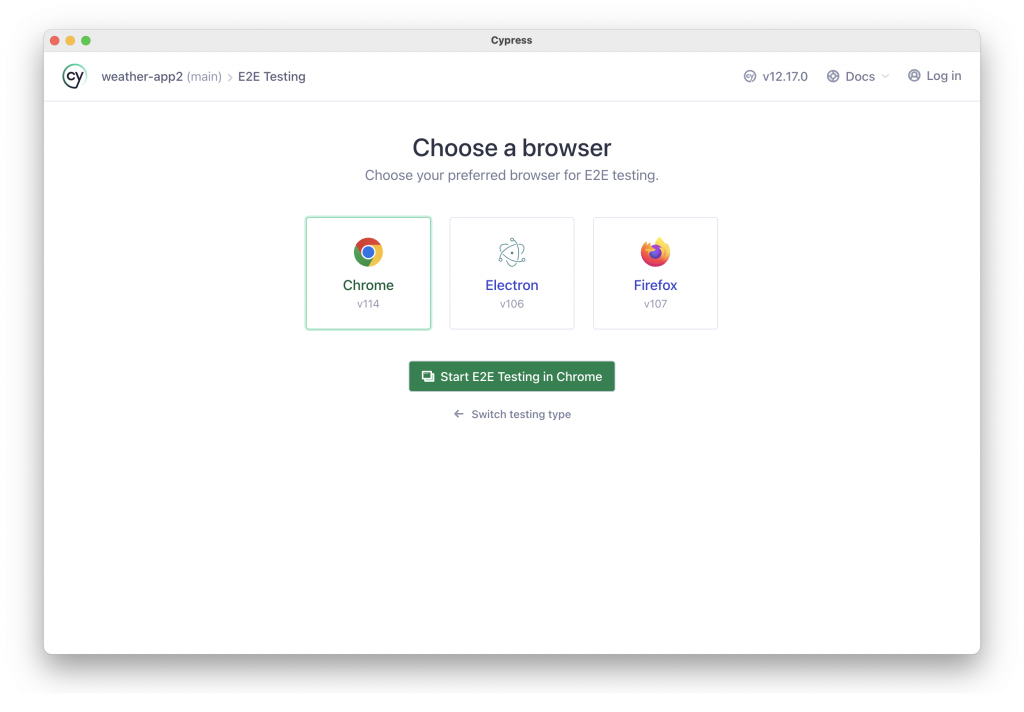
Cypress will create add several files including cypress.config.js
and a cypress/
directory. Here, you will add that new configuration and make a few more edits.
Then stop the cypress process in your Terminal window using Crtl-C before running the next script:
git add . && git commit -m "cypress config" && sed -i '' -e 's/ "cypress:open": "cypress open",//g' package.json && sed -i '' -e 's/ "cypress:run": "cypress run",/ "e2e": "start-server-and-test dev http:\/\/localhost:3000 \\"cypress open --e2e\\"",\n "e2e:headless": "start-server-and-test dev http:\/\/localhost:3000 \\"cypress run --e2e\\"",/g' package.json && git add . && git commit -m "cypress runner for nextjs" && sed -i '' -e 's/e2e: {/e2e: {\n baseUrl: "http:\/\/localhost:3000",\n/g' cypress.config.js && mkdir cypress/e2e && echo "describe('home page spec', () => {\n it('can load the home page', () => {\n cy.visit('/')\n cy.get('#__next').should('contain', \"Hello Next JS\")\n })\n})\n" >> cypress/e2e/homepage.cy.js && yarn add start-server-and-test && git add . && git commit -m "adds start-server-and-test, configures cypress for NextJS environment"
Run Cypress using yarn run e2e
. Choose E2E Testing and Chrome on the first and second window, respectively.
Then you will see the page that lists your Cypress tests. Click homepage.cy.js
to run the new spec. You should see the spec run and pass.
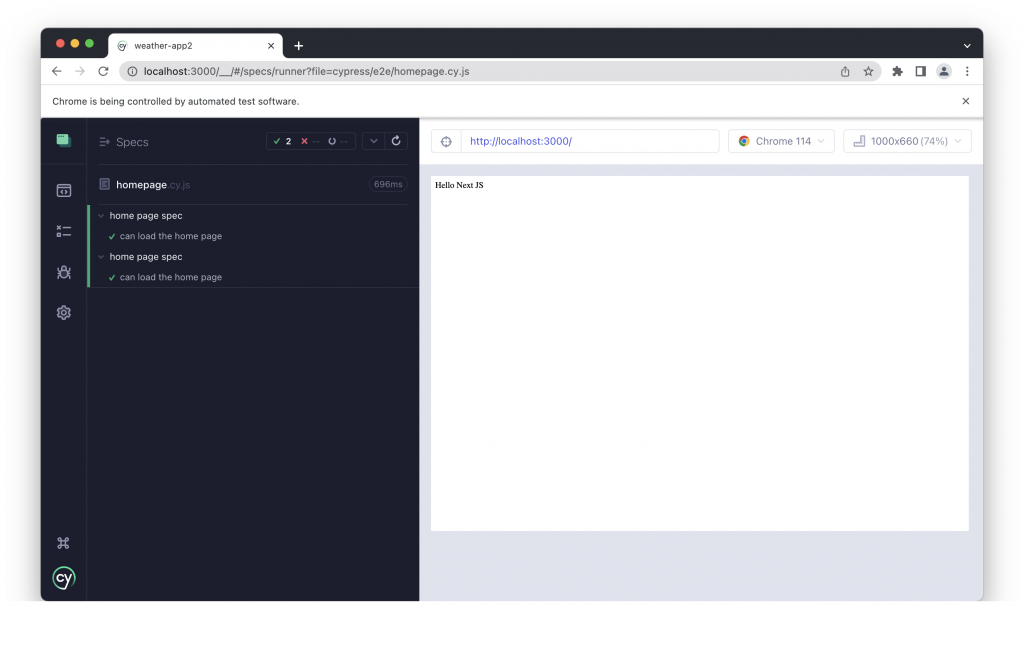