This is a companion blog post to my in-depth article Automated Testing fro 10,000
EXAMPLE 1 (Java)
Let’s make a quick Java project using:
mvn archetype:generate -DarchetypeGroupId=org.apache.maven.archetypes -DarchetypeArtifactId=maven-archetype-quickstart -DarchetypeVersion=1.4 -DgroupId=a -DartifactId=ExampleJUnitApp -Dversion=1 -Dpackage="widget"
(If you don’t have Maven installed on your computer, you must do that first.)
Open the newly created ExampleJUnitApp and navigate to test/java/widget/AppTest.jav
a
package widget;
import static org.junit.Assert.assertTrue;
import org.junit.Test;
/**
* Unit test for simple App.
*/
public class AppTest
{
/**
* Rigorous Test :-)
*/
@Test
public void shouldAnswerWithTrue()
{
assertTrue( true );
}
}
Here we meet our very first basic test. This test doesn’t even test any app code at all. All it does is says “assertTrue” against the static value true itself. That’s not very interesting.
Change the line assertTrue( true );
to assertTrue( false );
and use mvn test
to run the tests:
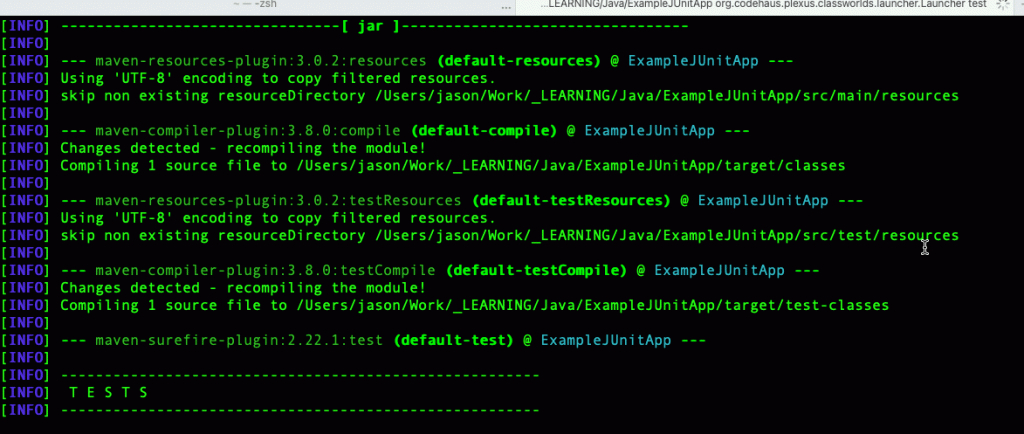
Now go back and change the test back to
assertTrue( true );
Re-run with mvn test
again. This time, we see “BUILD SUCCESS.”
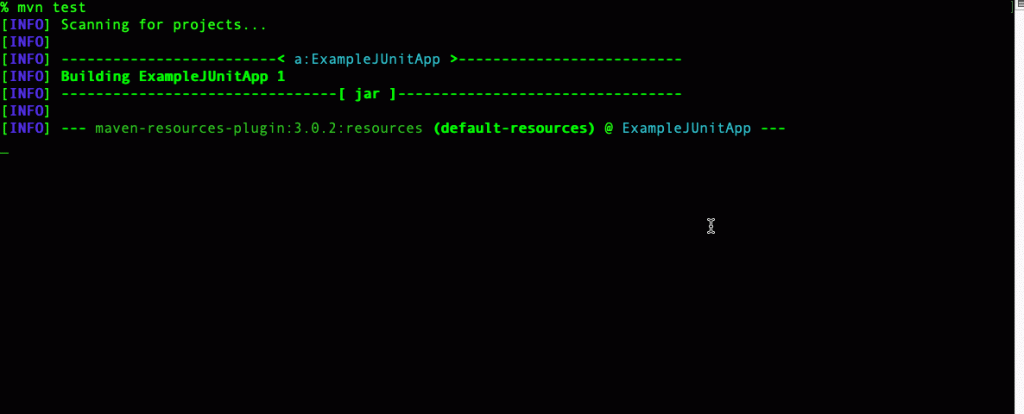
In this example, we didn’t actually implement anything or even look at our app code, but it gives you a demonstration of how TDD works: You iterate your tests until you see them “go green” or pass.